Intro
Master Cpp Calendar with 5 expert tips, including date manipulation, time zone handling, and scheduling. Boost productivity with C++ calendar management, date calculation, and event organization techniques.
The C++ programming language has been a cornerstone of software development for decades, and one of its most useful features is the ability to create and manipulate calendars. Calendars are essential in a wide range of applications, from simple reminders to complex scheduling systems. In this article, we will delve into the world of C++ calendar programming, exploring five valuable tips to help you create and manage calendars with ease.
C++ provides a robust set of libraries and tools for working with dates and times, including the ctime
library, which offers functions for manipulating dates and times. However, working with calendars can be complex, especially when dealing with different time zones, daylight saving time, and cultural variations. With the right techniques and libraries, you can create powerful and flexible calendar systems that meet your needs.
The importance of calendars in software development cannot be overstated. From scheduling appointments to tracking deadlines, calendars play a crucial role in many applications. By mastering the art of C++ calendar programming, you can create more efficient, effective, and user-friendly software systems. Whether you are a seasoned developer or just starting out, the tips and techniques presented in this article will help you take your calendar programming skills to the next level.
Understanding the C++ Date and Time Library
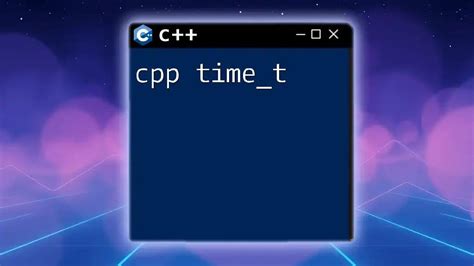
The C++ ctime
library provides a comprehensive set of functions for working with dates and times. This library includes functions for getting the current date and time, manipulating dates and times, and formatting output. To work effectively with calendars, you need to understand how to use these functions to create, manipulate, and display dates and times.
One of the key functions in the ctime
library is time()
, which returns the number of seconds since the Unix epoch (January 1, 1970, 00:00:00 UTC). You can use this function to get the current date and time, and then manipulate it using other functions in the library. For example, you can use the localtime()
function to convert the time to a struct tm
object, which contains members for the year, month, day, hour, minute, and second.
Using the time() Function
The `time()` function is a crucial part of the `ctime` library, and it's essential to understand how to use it effectively. Here are some key points to keep in mind: * The `time()` function returns the number of seconds since the Unix epoch. * You can use the `localtime()` function to convert the time to a `struct tm` object. * The `struct tm` object contains members for the year, month, day, hour, minute, and second.Working with Dates and Times in C++
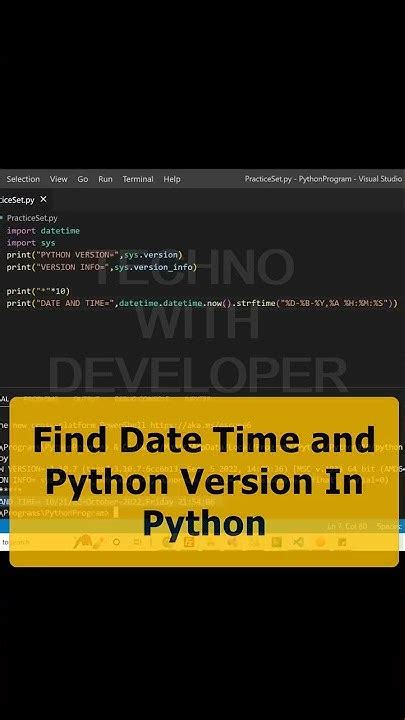
Working with dates and times in C++ can be complex, especially when dealing with different time zones and cultural variations. However, with the right techniques and libraries, you can create powerful and flexible calendar systems that meet your needs. Here are some key tips to keep in mind:
- Use the
ctime
library to get the current date and time, and to manipulate dates and times. - Use the
struct tm
object to work with dates and times in a structured way. - Be aware of the differences between local time and UTC time, and use the
localtime()
andgmtime()
functions accordingly.
Using the struct tm Object
The `struct tm` object is a crucial part of the `ctime` library, and it's essential to understand how to use it effectively. Here are some key points to keep in mind: * The `struct tm` object contains members for the year, month, day, hour, minute, and second. * You can use the `localtime()` function to convert the time to a `struct tm` object. * You can use the `mktime()` function to convert a `struct tm` object to a `time_t` object.Creating a Calendar Class in C++
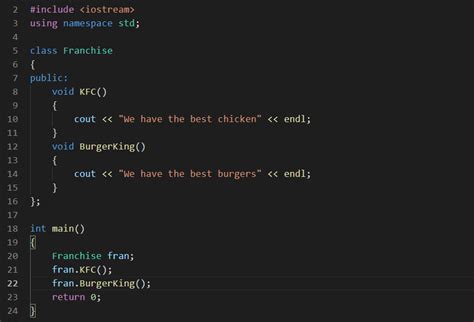
Creating a calendar class in C++ can be a powerful way to work with dates and times in a structured and object-oriented way. By encapsulating the data and behavior of a calendar in a single class, you can create a flexible and reusable calendar system that meets your needs. Here are some key tips to keep in mind:
- Use the
ctime
library to get the current date and time, and to manipulate dates and times. - Use the
struct tm
object to work with dates and times in a structured way. - Define a class that encapsulates the data and behavior of a calendar, including methods for getting and setting the date and time.
Defining the Calendar Class
Defining the calendar class is a crucial part of creating a calendar system in C++. Here are some key points to keep in mind: * Define a class that encapsulates the data and behavior of a calendar. * Include members for the year, month, day, hour, minute, and second. * Define methods for getting and setting the date and time, including constructors and destructors.Using the Calendar Class
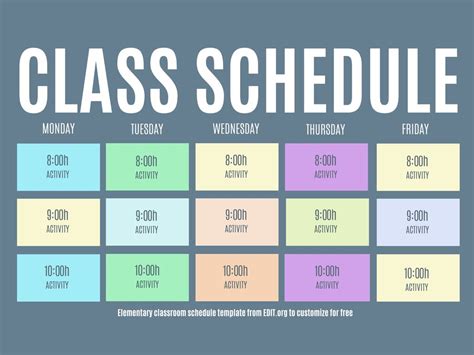
Using the calendar class is a crucial part of creating a calendar system in C++. By creating objects of the calendar class, you can work with dates and times in a structured and object-oriented way. Here are some key tips to keep in mind:
- Create objects of the calendar class to work with dates and times.
- Use the methods of the calendar class to get and set the date and time.
- Use the
ctime
library to manipulate dates and times, and to convert between different time zones.
Example Code
Here is an example of how to use the calendar class: ```cpp #includeclass Calendar { public: Calendar(int year, int month, int day, int hour, int minute, int second) { // Initialize the calendar object }
void setDate(int year, int month, int day, int hour, int minute, int second) {
// Set the date and time
}
void getDate(int& year, int& month, int& day, int& hour, int& minute, int& second) {
// Get the date and time
}
};
int main() { // Create a calendar object Calendar calendar(2022, 1, 1, 0, 0, 0);
// Get the date and time
int year, month, day, hour, minute, second;
calendar.getDate(year, month, day, hour, minute, second);
// Print the date and time
std::cout << "Date: " << year << "-" << month << "-" << day << " " << hour << ":" << minute << ":" << second << std::endl;
return 0;
}
Best Practices for C++ Calendar Programming
Best practices are essential for C++ calendar programming, as they help ensure that your code is efficient, effective, and easy to maintain. Here are some key tips to keep in mind:
* Use the `ctime` library to get the current date and time, and to manipulate dates and times.
* Use the `struct tm` object to work with dates and times in a structured way.
* Define a class that encapsulates the data and behavior of a calendar, including methods for getting and setting the date and time.
Using const Correctness
Using const correctness is a crucial part of C++ calendar programming, as it helps ensure that your code is efficient and effective. Here are some key points to keep in mind:
* Use const keywords to specify that a variable or function should not be modified.
* Use const references to pass variables to functions without modifying them.
* Use const pointers to point to variables without modifying them.
C++ Calendar Programming Image Gallery
What is the best way to handle time zones in C++ calendar programming?
+
The best way to handle time zones in C++ calendar programming is to use the `ctime` library and the `struct tm` object. You can use the `localtime()` function to convert the time to a `struct tm` object, and then use the `tm_zone` member to get the time zone.
How do I create a calendar class in C++?
+
To create a calendar class in C++, you can define a class that encapsulates the data and behavior of a calendar. You can include members for the year, month, day, hour, minute, and second, and define methods for getting and setting the date and time.
What are some best practices for C++ calendar programming?
+
Some best practices for C++ calendar programming include using the `ctime` library, using const correctness, and defining a class that encapsulates the data and behavior of a calendar. You should also use the `struct tm` object to work with dates and times in a structured way.
How do I handle daylight saving time in C++ calendar programming?
+
To handle daylight saving time in C++ calendar programming, you can use the `ctime` library and the `struct tm` object. You can use the `localtime()` function to convert the time to a `struct tm` object, and then use the `tm_isdst` member to get the daylight saving time flag.
What are some common pitfalls to avoid in C++ calendar programming?
+
Some common pitfalls to avoid in C++ calendar programming include not handling time zones correctly, not using const correctness, and not defining a class that encapsulates the data and behavior of a calendar. You should also avoid using outdated or deprecated functions and libraries.
In conclusion, C++ calendar programming is a complex and nuanced topic that requires careful consideration of many factors, including time zones, daylight saving time, and cultural variations. By following the tips and best practices outlined in this article, you can create powerful and flexible calendar systems that meet your needs. Whether you are a seasoned developer or just starting out, the techniques and libraries presented in this article will help you take your calendar programming skills to the next level. So why not start today and explore the world of C++ calendar programming? Share your thoughts and experiences in the comments below, and don't forget to share this article with your friends and colleagues who may be interested in learning more about this fascinating topic.