Intro
Create interactive Pyspark calendar heatmaps with visualization tools, leveraging data analysis and Spark SQL for insightful date-based patterns and trends discovery.
Pyspark is a powerful tool for data processing and analysis, and when combined with visualization libraries like Matplotlib and Seaborn, it can be used to create informative and engaging visualizations. One type of visualization that can be particularly useful for understanding patterns and trends in data is the calendar heatmap. In this article, we will explore how to create a calendar heatmap visualization using Pyspark.
Calendar heatmaps are a type of visualization that displays data on a calendar, with each day represented by a color that corresponds to the value of the data for that day. This type of visualization can be particularly useful for understanding patterns and trends in data that have a temporal component, such as website traffic, sales, or weather data. By using a calendar heatmap, we can quickly and easily see which days of the week or month are busiest, which seasons are most popular, and other patterns and trends that may be hidden in the data.
To create a calendar heatmap using Pyspark, we will need to start by loading our data into a Pyspark dataframe. This can be done using the spark.read
method, which allows us to load data from a variety of sources, including CSV files, JSON files, and databases. Once our data is loaded, we will need to preprocess it to ensure that it is in a format that can be used to create a calendar heatmap. This may involve converting date columns to a standard format, handling missing values, and aggregating data to the desired level of granularity.
Preprocessing Data for Calendar Heatmap
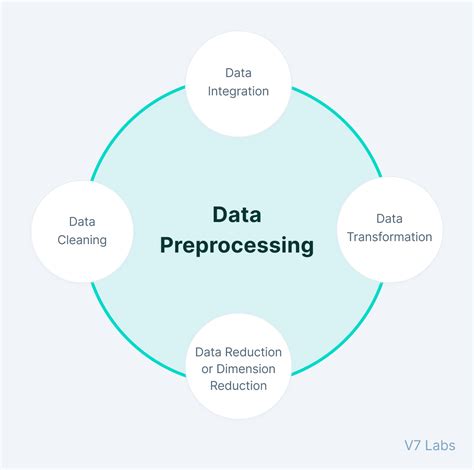
Once our data is preprocessed, we can use the pyspark.sql.functions
module to create a new column that represents the day of the week or month. This can be done using the dayofweek
or dayofmonth
functions, which return the day of the week or month for a given date. We can also use the month
or year
functions to extract the month or year from a date column.
Creating a Calendar Heatmap with Pyspark
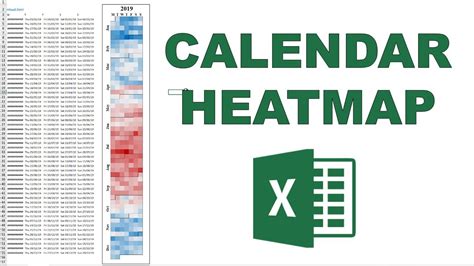
To create the calendar heatmap itself, we can use the matplotlib
library, which provides a wide range of visualization tools, including heatmaps. We will need to pivot our data to create a matrix that can be used to create the heatmap, with the days of the week or month on one axis and the months or years on the other axis. We can then use the imshow
function to create the heatmap, with the color of each cell corresponding to the value of the data for that day.
Example Code for Creating a Calendar Heatmap
```python from pyspark.sql import SparkSession import matplotlib.pyplot as plt import numpy as npCreate a SparkSession
spark = SparkSession.builder.appName("Calendar Heatmap").getOrCreate()
Load data into a Pyspark dataframe
data = spark.read.csv("data.csv", header=True, inferSchema=True)
Preprocess data
data = data.withColumn("dayofweek", dayofweek("date")) data = data.withColumn("month", month("date")) data = data.withColumn("year", year("date"))
Pivot data to create a matrix for the heatmap
pivoted_data = data.groupBy("dayofweek", "month", "year").count().pivot("dayofweek", [1, 2, 3, 4, 5, 6, 7]).sum("count")
Create a numpy array from the pivoted data
array = pivoted_data.toPandas().values
Create the heatmap
plt.imshow(array, cmap="hot", interpolation="nearest") plt.xlabel("Month") plt.ylabel("Day of Week") plt.title("Calendar Heatmap") plt.show()
Customizing the Calendar Heatmap
Once we have created the calendar heatmap, we can customize it to better suit our needs. This may involve changing the color scheme, adding labels or titles, or modifying the layout of the heatmap. We can also use other visualization libraries, such as Seaborn or Plotly, to create more complex and interactive visualizations.
Adding Interactive Elements to the Calendar Heatmap
We can add interactive elements to the calendar heatmap using libraries like Plotly or Bokeh. These libraries provide a range of tools for creating interactive visualizations, including hover text, zooming, and animations. By adding interactive elements to the calendar heatmap, we can make it more engaging and easier to use, and provide more insights into the data.
Using Calendar Heatmaps for Analysis and Decision-Making
Calendar heatmaps can be a powerful tool for analysis and decision-making, particularly when working with temporal data. By using a calendar heatmap, we can quickly and easily identify patterns and trends in the data, and gain insights into how the data changes over time. This can be particularly useful in a variety of applications, including marketing, finance, and healthcare.
Example Use Cases for Calendar Heatmaps
* Identifying peak seasons or periods of high demand
* Analyzing website traffic or user engagement
* Understanding patterns in sales or revenue
* Visualizing weather or climate data
* Identifying trends in healthcare or disease outbreaks
Calendar Heatmap Image Gallery
What is a calendar heatmap?
+
A calendar heatmap is a type of visualization that displays data on a calendar, with each day represented by a color that corresponds to the value of the data for that day.
How do I create a calendar heatmap using Pyspark?
+
To create a calendar heatmap using Pyspark, you will need to load your data into a Pyspark dataframe, preprocess the data, pivot the data to create a matrix for the heatmap, and then use a visualization library like Matplotlib or Seaborn to create the heatmap.
What are some common use cases for calendar heatmaps?
+
Calendar heatmaps can be used in a variety of applications, including marketing, finance, and healthcare. Some common use cases include identifying peak seasons or periods of high demand, analyzing website traffic or user engagement, and understanding patterns in sales or revenue.
How can I customize my calendar heatmap?
+
You can customize your calendar heatmap by changing the color scheme, adding labels or titles, or modifying the layout of the heatmap. You can also use other visualization libraries, such as Seaborn or Plotly, to create more complex and interactive visualizations.
What are some benefits of using calendar heatmaps?
+
Calendar heatmaps can be a powerful tool for analysis and decision-making, particularly when working with temporal data. They can help you quickly and easily identify patterns and trends in the data, and gain insights into how the data changes over time.
We hope this article has provided you with a comprehensive overview of how to create a calendar heatmap using Pyspark. By following the steps outlined in this article, you can create your own calendar heatmap and gain insights into your data. If you have any questions or need further assistance, please don't hesitate to reach out. We encourage you to share this article with others who may be interested in learning more about calendar heatmaps and Pyspark. Additionally, we invite you to comment below and let us know how you plan to use calendar heatmaps in your own work.